admin 管理员组文章数量: 900433
一.Pdf文件格式实现预览
方法一:
第一步:安装
npm i pdfh5
第二步:使用
<template>
<div class="pdf" v-show="visible">
<div @click="visible = false"></div>
<div id="preViewPdf"></div>
</div>
</template>
<script>
import Pdfh5 from 'pdfh5';
import 'pdfh5/css/pdfh5.css';
export default {
name: 'pdfH5',
data() {
return {
visible: false,
pdfh5: null,
};
},
methods: {
openPdf(url) {
this.visible = true;
//若是在项目中需要后端把文件的正确路径返回,可以浏览器上测试是否能打开,若能,那通过以下方法可实现预览
this.pdfh5 = new Pdfh5('#preViewPdf', {
pdfurl: url,
});
},
},
created(){
let path =
'http://celiang.oss-cn-hangzhou.aliyuncs/measurement/2022-01/18/75it6phpocqYFV1642488558220118.pdf';
this.openPdf(path)
}
};
</script>
<style lang="less" scoped>
.pdf {
position: fixed;
top: 0;
left: 0;
width: 100vw;
height: 100vh;
background: #000;
overflow: hidden;
z-index: 200;
}
</style>
方法二:
第一步:安装
npm install vue-pdf
第二步:使用
<template>
<div>
<!-- 预览PDF -->
<van-dialog v-model="showDialog">
<template>
<div>
<div class="tools">
<van-button
:theme="'default'"
type="submit"
:title="'上一页'"
@click.stop="prePage"
class="mr10"
>
上一页</van-button
>
<van-button
:theme="'default'"
type="submit"
:title="'下一页'"
@click.stop="nextPage"
class="mr10"
>
下一页</van-button
>
<div class="page">{{ pageNum }}/{{ pageTotalNum }}</div>
<van-button
:theme="'default'"
type="submit"
:title="'顺时针旋转'"
@click.stop="clock"
class="mr10"
>
顺时针旋转</van-button
>
<van-button
:theme="'default'"
type="submit"
:title="'逆时针旋转'"
@click.stop="counterClock"
class="mr10"
>
逆时针旋转</van-button
>
<van-button
:theme="'default'"
type="submit"
:title="'打印'"
@click.stop="pdfPrintAll"
class="mr10"
>
打印</van-button
>
</div>
<pdf
ref="pdf"
:src="url"
:page="pageNum"
:rotate="pageRotate"
@progress="loadedRatio = $event"
@page-loaded="pageLoaded($event)"
@num-pages="pageTotalNum = $event"
@error="pdfError($event)"
@link-clicked="page = $event"
></pdf>
</div>
</template>
</van-dialog>
</div>
</template>
<script>
import pdf from 'vue-pdf';
export default {
components: {
pdf,
},
props: {
isShowDialog: {
type: Boolean,
default: false,
},
},
watch: {
isShowDialog(res) {
this.showDialog = res;
},
},
data() {
return {
showDialog: false,
url: 'http://storage.xuetangx/public_assets/xuetangx/PDF/PlayerAPI_v1.0.6.pdf',
pageNum: 1,
pageTotalNum: 1,
pageRotate: 0,
// 加载进度
loadedRatio: 0,
curPageNum: 0,
};
},
methods: {
/**
* 预览PDF
*/
previewPDF() {
this.showDialog = true;
},
// 上一页函数,
prePage() {
var page = this.pageNum;
if (page >= 1) {
this.pageNum = page;
console.log('aaa', page);
} else if (page <= this.pageTotalNum) {
this.pageNum = this.pageTotalNum - 1;
}
},
// 下一页函数
nextPage() {
var page = this.pageNum;
if (page + 1 <= this.pageTotalNum) {
page = page + 1;
}
this.pageNum = page;
},
// 页面顺时针翻转90度。
clock() {
this.pageRotate += 90;
},
// 页面逆时针翻转90度。
counterClock() {
this.pageRotate -= 90;
},
// 页面加载回调函数,其中e为当前页数
pageLoaded(e) {
if (e) {
this.curPageNum = e;
}
},
// 错误时回调函数。
pdfError(error) {
console.error(error);
},
// 打印全部
pdfPrintAll() {
this.$refs.pdf.print();
},
},
created(){
this.previewPDF()
}
};
</script>
二.docx文件格式实现预览
只能实现docx文件类型,doc文件类型不支持
第一步:安装
npm i docx-preview
第二步:使用
<template>
<div>
<div ref="files" id="files"></div>
<!-- 本地预览word可行 -->
<div class="my-component" ref="preview">
<input type="file" @change="preview" ref="file" />
</div>
</div>
</template>
<script>
const docx = require('docx-preview');
window.JSZip = require('jszip');
export default {
methods: {
//通过本地上传的附件进行预览
//若是在项目中,需要后端把文件流返回给前端,前端拿到二进制流之后转换成blob即可实现预览
//如:let blob=new Blob([res],{type:"application/octet-stream;chartset=UTF-8"})
/**preview() {
this.$nextTick(()=>{
docx.renderAsync(blob, this.$refs.preview);
})
}**/
preview() {
docx.renderAsync(this.$refs.file.files[0], this.$refs.preview);
},
//通过网上找的地址也可实现文件预览,
getDocCode() {
window.open(
'https://view.xdocin/view?src=' +
encodeURIComponent('https://view.xdocin/demo/view.docx')
);
}
},
created(){
this.getDocCode()
}
};
</script>
三.xlsx文件格式实现预览
只能实现xlsx文件类型,xls文件类型不支持
第一步:安装
npm install luckyexcel
第二步:使用
<template>
<div class="hello">
<div
id="mysheet"
style="margin: 0px; padding: 0px; width: 100%; height: 100vh"
></div>
</div>
</template>
<script>
import LuckyExcel from 'luckyexcel';
//1.如果是在项目中使用,可能会报错,需要在index.html中通过csdn把所有依赖引入进来才能使用,2.如果实现不行,也可以通过把文件下载下来,把它放到一个文件夹中,在index.html中引入使用(此方法亲测有效)如下图是下载好的文件
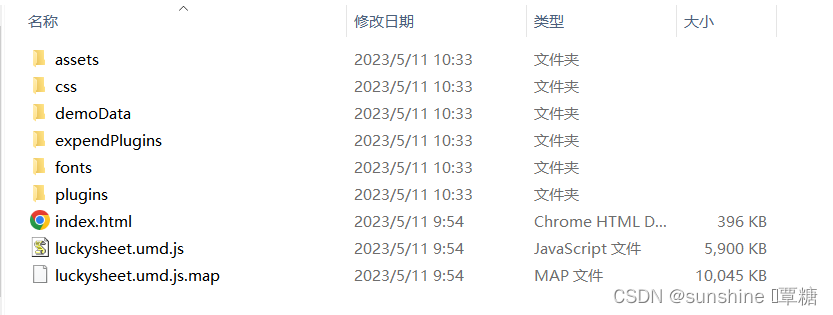
export default {
name: 'vueSheet',
methods: {
//通过插件实现预览
//若是在项目中,需要后端把文件流返回给前端,前端拿到二进制流之后转换成blob即可实现预览
//如:let file=new File([res],{type:"application/vnd.ms-excel;chartset=utf-8"})
/**getExcel() {
LuckyExcel.transformExcelToLuckyByUrl(file,(exportJson)=>{})
}
**/
getExcel() {
LuckyExcel.transformExcelToLuckyByUrl(
'http://celiang.oss-cn-hangzhou.aliyuncs/measurement/2022-05/30/zG4ZPphpTiDPkG1653875854220530.xlsm',
'',
(exportJson) => {
if (exportJson.sheets == null || exportJson.sheets.length == 0) {
alert('文件读取失败!');
return;
}
console.log('预览', window.luckysheet);
// 销毁原来的表格
window.luckysheet.destroy();
// 重新创建新表格
window.luckysheet.create({
container: 'mysheet', // 设定DOM容器的id
showtoolbar: false, // 是否显示工具栏
showinfobar: false, // 是否显示顶部信息栏
showstatisticBar: false, // 是否显示底部计数栏
sheetBottomConfig: false, // sheet页下方的添加行按钮和回到顶部按钮配置
allowEdit: false, // 是否允许前台编辑
enableAddRow: false, // 是否允许增加行
enableAddCol: false, // 是否允许增加列
sheetFormulaBar: false, // 是否显示公式栏
enableAddBackTop: false, //返回头部按钮
data: exportJson.sheets, //表格内容
title: exportJson.info.name, //表格标题
});
}
);
},
//通过在线文件预览的方法
getFile() {
//方法可行,但是文件类型不对
/* let docUrl =
'http://celiang.oss-cn-hangzhou.aliyuncs/measurement/2022-05/30/zG4ZPphpTiDPkG1653875854220530.xlsm';
let url = encodeURIComponent(docUrl);
let officeUrl = 'http://view.officeapps.live/op/view.aspx?src=' + url;
window.open(officeUrl, '_target'); */
//此方法可行
window.open(
'https://view.xdocin/view?src=' +
encodeURIComponent(
'http://celiang.oss-cn-hangzhou.aliyuncs/measurement/2022-05/30/zG4ZPphpTiDPkG1653875854220530.xlsm'
)
);
},
},
created() {
this.getExcel()
//this.getFile()
},
};
</script>
版权声明:本文标题:App如何实现pdf,word,excel格式的文件预览? 内容由网友自发贡献,该文观点仅代表作者本人, 转载请联系作者并注明出处:http://www.freenas.com.cn/jishu/1726311843h934550.html, 本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。如发现本站有涉嫌抄袭侵权/违法违规的内容,一经查实,本站将立刻删除。
发表评论